Build a REST API in Four Simple Steps
Learn how to build a REST API in four simple steps. Install Node.js, initialize the project, create an empty JavaScript file, and install Express. Then, you'll be ready to create your API.

Pratham
DevRel @HyperspaceAI โข Prev @Rapid_API โข Partners @iteration_x @10Web_io @StockImgAI @JamDotDev @CohesiveAI @refine_dev @chatlingai @Inboxproio @Docus_ai
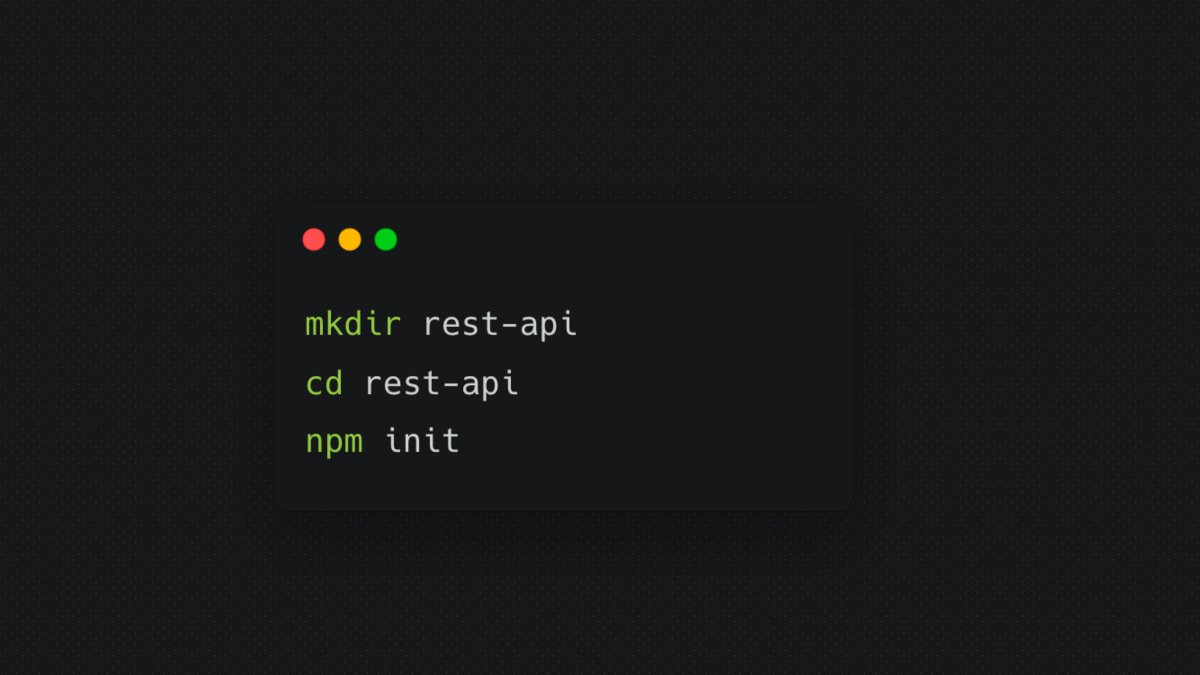
-
Build a REST API in four simple steps.
— Pratham (@Prathkum) June 19, 2023
Thread ๐งต -
Step 1: Install Node.js
— Pratham (@Prathkum) June 19, 2023
I'm assuming you have Node.js installed on your machine. If you haven't installed it, click on the following link and install it simply.https://t.co/xKueCxRAUd -
Step 2: Initialize the project
— Pratham (@Prathkum) June 19, 2023
Let's start; create an empty directory and initialize your project by running the following command. pic.twitter.com/5m6Da2qFpW -
The next step is to create an empty JavaScript file (File name could be anything, in this case, it's index.js) and install Express.
— Pratham (@Prathkum) June 19, 2023
๐ https://t.co/Yw1oLDvoOc
Express is a minimal and easy-to-learn framework for Node. pic.twitter.com/dXPa2KSZq1 -
Step 3: Starting the server
— Pratham (@Prathkum) June 19, 2023
Now that everything is ready, it's time to code our actual API.
Let's start the server first.
In the below code snippet, we are initializing our `app` and starting the local server at port 3000.
Run `node index.js` to start the server. pic.twitter.com/w9qf3M5uPZ -
For more context, listen() function is used to establish the connection on specified host and port.
— Pratham (@Prathkum) June 19, 2023
It takes two params:
- The first is the port number or host.
- The second (optional) is a callback function that runs after listening to a specified host or value. -
Moving forward, let's try to access "localhost:3000" in the browser and see what we are getting.
— Pratham (@Prathkum) June 19, 2023
We are getting a "404 Not Found" response which is correct as we haven't defined any endpoints yet. pic.twitter.com/4vI4PXeiBS -
I prefer RapidAPI Client for VS Code extension to test and build API in VS Code.
— Pratham (@Prathkum) June 19, 2023
You can for other options as well like Postman, Thunder Client, etc.
Go ahead and install it.
๐ https://t.co/FR0QhyTi8v pic.twitter.com/Rd8CCZntkv -
As now our server is running successfully at port 3000, let's create an endpoint.
— Pratham (@Prathkum) June 19, 2023 -
Step 4: Create Endpoints
— Pratham (@Prathkum) June 19, 2023
The `get` method allows us to create HTTP GET requests.
It accepts two params:
- The first is path/route.
- The second is a callback function that handles the request to the specified route. pic.twitter.com/3OQaqjv3ce -
The callback function itself accepts two arguments:
— Pratham (@Prathkum) June 19, 2023
- The first is the request which is the data to the server.
- The second is the response which is the data to the client. pic.twitter.com/nqNeIT9Vmh -
Suppose you want to display all the users whenever the client requests the "/users" endpoint.
— Pratham (@Prathkum) June 19, 2023
To return the data from the server to the client, we have the `send` method.
In the code snippet below, we send an array of objects with `name` and `id` fields. pic.twitter.com/BiuW1F7zUs -
Perfect!
— Pratham (@Prathkum) June 19, 2023
Let's restart the server by running the `node index.js` command and see what we are getting. pic.twitter.com/ymAS8GPOAT -
You can make as many endpoints as you want using the same technique.
— Pratham (@Prathkum) June 19, 2023
For demonstration purposes, let's quickly create a POST request endpoint. -
As POST request is to create or add new data to the server. We first need to add middleware so that we can parse the JSON body. pic.twitter.com/Isvcq56QJt
— Pratham (@Prathkum) June 19, 2023 -
We are defining a POST endpoint at the "/user/3" route.
— Pratham (@Prathkum) June 19, 2023
We implemented the logic of throwing a "400 Bad Request" status code if the user forgets to pass the name value in the request body. pic.twitter.com/aNtj6ujYkL -
Let's try to access this endpoint now. pic.twitter.com/BGYNeKNNe5
— Pratham (@Prathkum) June 19, 2023 -
As you can see, we are getting a response. ๐
— Pratham (@Prathkum) June 19, 2023
Great! We have just built a REST API. -
With that said, that's pretty much it for this thread.
— Pratham (@Prathkum) June 19, 2023
Follow @PrathKum for more exciting content such as this one.